How to Get Japan's Treasury Interest Rates Retrieved in Pandas
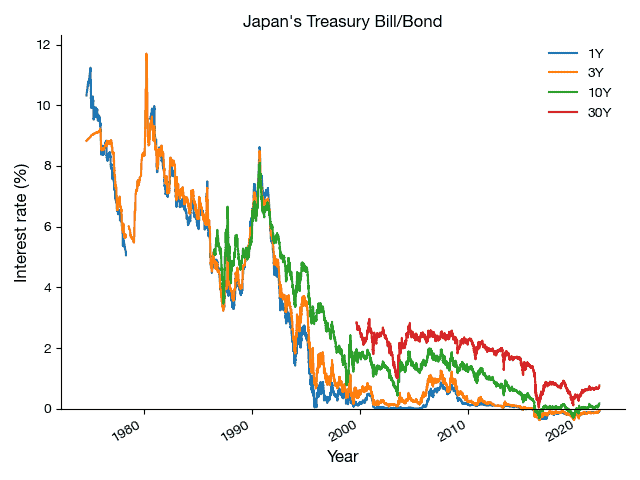
Steps
The Ministry of Finance provides the historical data of Japan's Treasury interest rates here.
https://www.mof.go.jp/english/policy/jgbs/reference/interest_rate/historical/jgbcme_all.csv
Interest Rate,,,,,,,,,,,,,,,(Unit : %)
Date,1Y,2Y,3Y,4Y,5Y,6Y,7Y,8Y,9Y,10Y,15Y,20Y,25Y,30Y,40Y
1974/9/24,10.327,9.362,8.83,8.515,8.348,8.29,8.24,8.121,8.127,-,-,-,-,-,-
1974/9/25,10.333,9.364,8.831,8.516,8.348,8.29,8.24,8.121,8.127,-,-,-,-,-,-
1974/9/26,10.34,9.366,8.832,8.516,8.348,8.29,8.24,8.122,8.128,-,-,-,-,-,-
1974/9/27,10.347,9.367,8.833,8.517,8.349,8.29,8.24,8.122,8.128,-,-,-,-,-,-
You can import and convert the data directly into a dataframe using Pandas' read_csv function. Since there are three digits below the decimal point and interest rates seem unlikely to have exceeded 100% in the last half-century in Japan, five significant figures will be sufficient, and thus I can safely set the dtype as np.float16.
import pandas as pd
import numpy as np
url="https://www.mof.go.jp/english/policy/jgbs/reference/interest_rate/historical/jgbcme_all.csv"
df = pd.read_csv(
url,
header=1,
dtype=np.float16,
index_col="Date",
na_values="-",
parse_dates=True
)
df.head(4)
1Y | 2Y | 3Y | 4Y | ... | |
Date | |||||
1974-09-24 | 10.327 | 9.362 | 8.830 | 8.515 | ... |
1974-09-25 | 10.333 | 9.364 | 8.831 | 8.516 | ... |
1974-09-26 | 10.340 | 9.366 | 8.832 | 8.516 | ... |
1974-09-27 | 10.347 | 9.367 | 8.833 | 8.517 | ... |
Here is the code for the chart above.
import matplotlib
from matplotlib import pyplot as plt
from matplotlib import rcParams
matplotlib.style.use("default")
rcParams["legend.frameon"] = False
rcParams["axes.spines.top"] = False
rcParams["axes.spines.right"] = False
rcParams["axes.labelsize"] = "large"
rcParams["font.sans-serif"] = ["Helvetica Neue"]
fig = plt.figure()
ax = fig.add_subplot()
df[["1Y", "3Y", "10Y", "30Y"]].plot(
ax=ax, xlabel="Year", ylabel="Interest rate (%)", clip_on=False
)
ax.set_ylim(0, ax.get_ylim()[1])
ax.set_title("Japan's Treasury Bills and Bonds")